During application development, we should consider logically correct and incorrect code execution based on the end-users requests, or the data posted by them. Sometimes even after testing our code using various test-cases, our code may not respond correctly to the request posted by end-users. Taking view of this reality, we implement our code via exception handling techniques using try-catch-finally block. This block successfully executes for all possible known types of exceptions e.g. File IO Read/Write issues, database connectivity issues etc. But what if during a request processing, a request fails due to the resource requested not available, or data posted by the end-user fails against some business rules. In this case, a web developer has to implement custom exception handling logic for handling such exceptions. This logic should be so flexible that whenever at any point in the application, such an exception occurs, this logic should be executed and exception handled and logged for further analysis.
Since ASP.NET MVC sits on top of the ASP.NET Runtime, it means that an MVC application can use ASP.NET's Application_Error event. In this case, any unhandled exception by MVC can be handled by the Application_Error event of ASP.NET. This is a great approach to handle Application Level Exceptions. But the problem of such an approach is that since it is at the application level and provides a lesser specific way of handling an exception, the MVC Controller Context data is lost. This means that we are no more handling an exception in our MVC application. However our requirement here is that we need to handle all exceptions at the MVC level only.
There are some simpler ways provided in MVC for handling exception. Please visit http://www.dotnetcurry.com/aspnet-mvc/1068/aspnet-mvc-exception-handling to learn more about it.
Section 1: Action Filter Lifecycle
In ASP.NET MVC, we have ActionFilter using which we can evaluate incoming requests and process them against these filters e.g. Authentication, Exceptions, Results etc. We can use Exception Filter to monitor any exceptions that occurs during MVC Request processing. In MVC, a request is accepted over the Controller, and then the ActionMethod in the controller is executed. The Exception ActionFilter is applied here. This Action Filter is executed within the scope of Action Method Execution. In the following diagram, the lifecycle of the ActionFilter for handing Exceptions is explained.
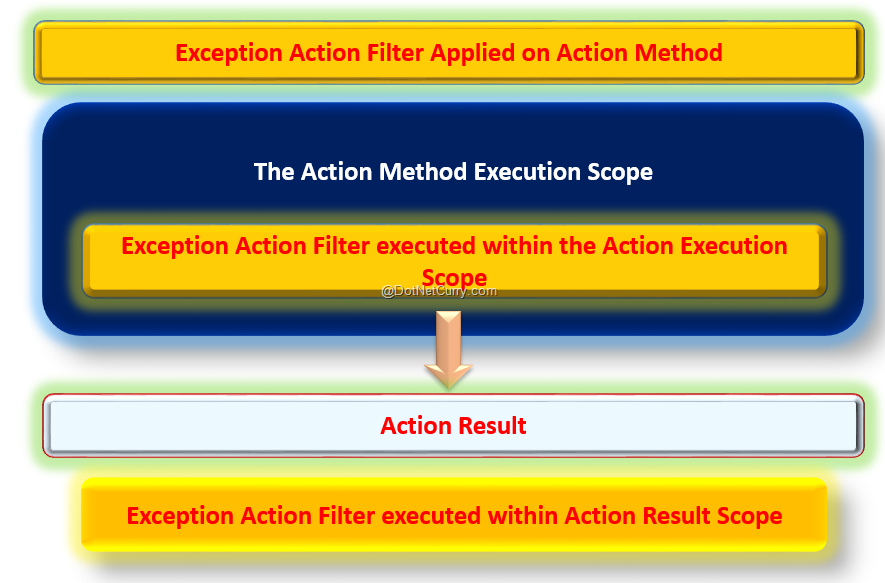
The exception filter is applied on the Action Method (alternatively we can even apply it on the Controller containing the Action Method.) The exception filter is executed within the action execution scope. If action method is executed successfully, then the Action Result will be generated where there may be a possibility of exception occurrence e.g. we are using custom view but it is not executed successfully for the current request.
We can implement a custom exception filter as per the needs of the application. Please visit http://devcurry.com/2012/08/custom-action-filter-in-aspnet-mvc-3.html to learn more about it. Furthermore, in Line of Business (LOB) apps, it is important not only to handle exceptions, but to log them in a persistent store (e.g. database) and mail the exception message details to the responsible authority. This makes our MVC application more maintainable.
In this article, we will implement a custom exception and we will use database table to log all exception message details.
Section 2 – Logging Exceptions in an ASP.NET MVC application
We will be implementing this application using Visual Studio 2015. You can use VS 2013 too.
Step 1: Open Visual Studio and create a new ASP.NET Web Application using New Project window. Set the name of the application as MVC_LoggingExcepion_with_ExceptionFilter. Clicking OK on this window will bring up the New ASP.NET Project window. Select Empty MVC project template as shown in the following image
Step 2: In the project, add a new Sql Server Database of name ApplicationDB.mdf. Leave this database blank. We will use Entity Framework Code first architecture.
Step 3: In the project in the Models folder, right-click and add a new ADO.NET Entity Data Model of name ApplicationModel. This will start the wizard, in this wizard, in the Choose Model Contents window, select Code First from Database, as shown in the following image
Click on the Next button and select ApplicationDB.mdf from this window. Click on finish, this will generate ApplicationModel class derived from DbContext class.
Step 4: In the Model folder, add a new class file of name EmployeeInfo.cs with following code in it:
using System.ComponentModel.DataAnnotations; namespace MVC_LoggingExcepion_with_ExceptionFilter.Models { public class EmployeeInfo { [Key] public int EmpNo { get; set; } public string EmpName { get; set; } public string Designation { get; set; } public decimal Salary { get; set; } } }
This is an entity class. We will use this for creating EmployeeInfo table using Code-First with Database approach. In the above class EmpNo is an attribute as Key so that it will be treated as primary key.
Step 5: Since the article describes about logging exception in database, we need a table to store log information. In the Models folder, add a new class file of name ExceptionLogger.cs with the following code in it.
using System; using System.ComponentModel.DataAnnotations; namespace MVC_LoggingExcepion_with_ExceptionFilter.Models { public class ExceptionLogger { [Key] public int Id { get; set; } public string ExceptionMessage { get; set; } public string ControllerName{ get; set; } public string ExceptionStackTrace { get; set; } public DateTime LogTime { get; set; } } }
The above class contains properties for storing exception details.
Step 6: In the ApplicationModel.cs class file, add the following properties in the ApplicationModel class (highlighted)
public partial class ApplicationModel : DbContext { public ApplicationModel() : base("name=ApplicationModel") { } public DbSet<EmployeeInfo> Employees { get; set; } public DbSet<ExceptionLogger> ExceptionLoggers{ get; set; } protected override void OnModelCreating(DbModelBuilder modelBuilder) { } }
These properties will update the database with Employees and ExceptionLoggers table.
Step 7: In the project, add a new folder of name CustomFilter. In this folder add a new class of name ExceptionHandlerAttribute with following code.
using MVC_LoggingExcepion_with_ExceptionFilter.Models; using System; using System.Web.Mvc; namespace MVC_LoggingExcepion_with_ExceptionFilter.CustomFilter { public class ExceptionHandlerAttribute : FilterAttribute, IExceptionFilter { public void OnException(ExceptionContext filterContext) { if (!filterContext.ExceptionHandled) { ExceptionLogger logger = new ExceptionLogger() { ExceptionMessage = filterContext.Exception.Message, ExceptionStackTrace = filterContext.Exception.StackTrace, ControllerName = filterContext.RouteData.Values["controller"].ToString(), LogTime = DateTime.Now }; ApplicationModel ctx = new ApplicationModel(); ctx.ExceptionLoggers.Add(logger); ctx.SaveChanges(); filterContext.ExceptionHandled = true; } } } }
The above class is derived from the FilterAttribute class, this means that the ExceptionHandlerAttribute class will be used as ActionFilter in the MVC Filter Attribute lifecycle as explained in Section 1. The class implements IExceptionFilter interface and its OnException() method. This method contains logic for storing exception information in ExceptionLoggers table of the database.
Step 8: We need to register the exception filter in our MVC application. To complete the registration, add a new class file of the name FilterConfig in the App_Start folder. In this class, add the following code
using MVC_LoggingExcepion_with_ExceptionFilter.CustomFilter; using System.Web.Mvc; namespace MVC_LoggingExcepion_with_ExceptionFilter.App_Start { public class FilterConfig { public static void RegisterGlobalFilters(GlobalFilterCollection filters) { filters.Add(new ExceptionHandlerAttribute()); } } }
Note: Since we have selected an Empty MVC template in Step 1 the above class is not present with us, but if you selected a readymade MVC template then the above class will be already present in the application. This class is used to add all Action Filters in the global configuration. This will be instantiated when the Web application starts.
In Global.asax, add the following line in the Application_Start. (highlighted).
protected void Application_Start() { AreaRegistration.RegisterAllAreas(); FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters); RouteConfig.RegisterRoutes(RouteTable.Routes); }
Step 8: In the Controllers folder, add a new controller of name EmployeeController with following action methods
using MVC_LoggingExcepion_with_ExceptionFilter.CustomFilter; using MVC_LoggingExcepion_with_ExceptionFilter.Models; using System; using System.Linq; using System.Web.Mvc; namespace MVC_LoggingExcepion_with_ExceptionFilter.Controllers { public class EmployeeController : Controller { ApplicationModel ctx = new ApplicationModel(); // GET: Employee public ActionResult Index() { var Emps = ctx.Employees.ToList(); return View(Emps); } public ActionResult Create() { return View(new EmployeeInfo()); } [ExceptionHandler] [HttpPost] public ActionResult Create(EmployeeInfo Emp) { if (Emp.Designation == "Manager" && (Emp.Salary < 40000 || Emp.Salary > 80000)) { throw new Exception("Salary is not Matching with Manager Designatgion"); } else if (Emp.Designation == "Operator" && (Emp.Salary < 10000 || Emp.Salary > 20000)) { throw new Exception("Salary is not Matching with Operator Designatgion"); } else { ctx.Employees.Add(Emp); ctx.SaveChanges(); } return View(Emp); } } }
The Index method returns all Employees. The Create with HttpPost method accepts an Employee object. This method contains some business rules on the Employee Salary based on the Designation. If these rules fail, then an Exception will be thrown. If the Employee Data is correct as per the business rules, then it will be saved in Employee table.
Step 9: Scaffold an Index and Create Views from Index and Create Action methods. In the RouteConfig.cs, modify the route expression as shown in the following code (highlighted)
public static void RegisterRoutes(RouteCollection routes) { routes.IgnoreRoute("{resource}.axd/{*pathInfo}"); routes.MapRoute( name: "Default", url: "{controller}/{action}/{id}", defaults: new { controller = "Employee", action = "Index", id = UrlParameter.Optional } ); }
The above code will run the Index action method of the EmployeeController which will display Index view.
Step 10: Apply breakpoints on the Create with HttpPost method of the EmployeeController class and the OnException method of the ExceptionHandlerAttribute class. Run the application, the Index View will be displayed. Click on Create New link on this view to display the Create View. Enter data in the Create view as shown in the following image
We have the Designation as Operator and Salary as 8666, click on the Create button, the control will enter in debug Mode, the if condition for the Operator will throw an exception as shown in the following image
Press F5, the control will enter ExceptionHandlerAttribute where the Database logging will take place. Close the application, the database table ExceptionLoggers will show the logged message as shown in the following image

Conclusion:
In a web application, Exception handling and logging is very important. ASP.NET MVC provides this facility using Exception Action Filters. We can create custom action filters to log these exception as per our business need.
0 comments :
Post a Comment